Notes and Discussion
Notes on critical days and project completion
I can’t say enough about Paul Hudson and his awesome site, hackingwithswift.com, that teaches Swift. I’ve bought tutorials and tried to learn from the manual (which is quite good), but I find Paul’s clear presentation of the information keeps me the most engaged and moving forward with my learning.
Day 22
End of project 2
This project called Guess the Flag was also used on the other 100 day challenge and I completed it then, but with SwiftUI it was so much more pleasing and satisfying to work on.
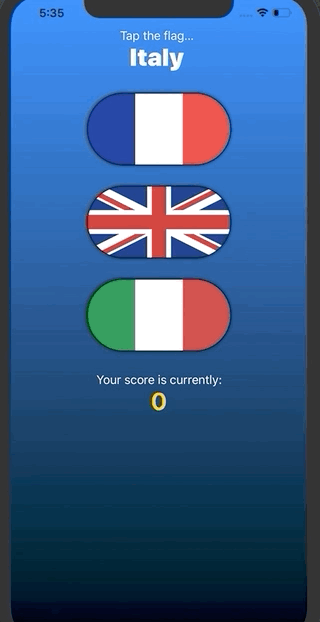
The project introduced more advanced things like Alerts and how to get them to trigger. The’re still a bit complex to run, but not too difficult. The challenges turned out to be simple this time around. Keeping score was as easy as creating a State property and referencing it in the Alert and when the user scored correctly. I also modified the code to ignore the answer if they were wrong and the score was 0. I don’t want them to dig themselves into a hole.
func flagTapped(_ number: Int){
if number == correctAnswer {
scoreTitle = "Correct"
self.score += 1
} else {
scoreTitle = "Wrong that's the flag for (countries[number])"
self.score -= (self.score == 0) ? 0 : 1
}
showingScore = true
}
Day 19
The first challenge where we were asked to create an app on our own using what we’ve learned so far.
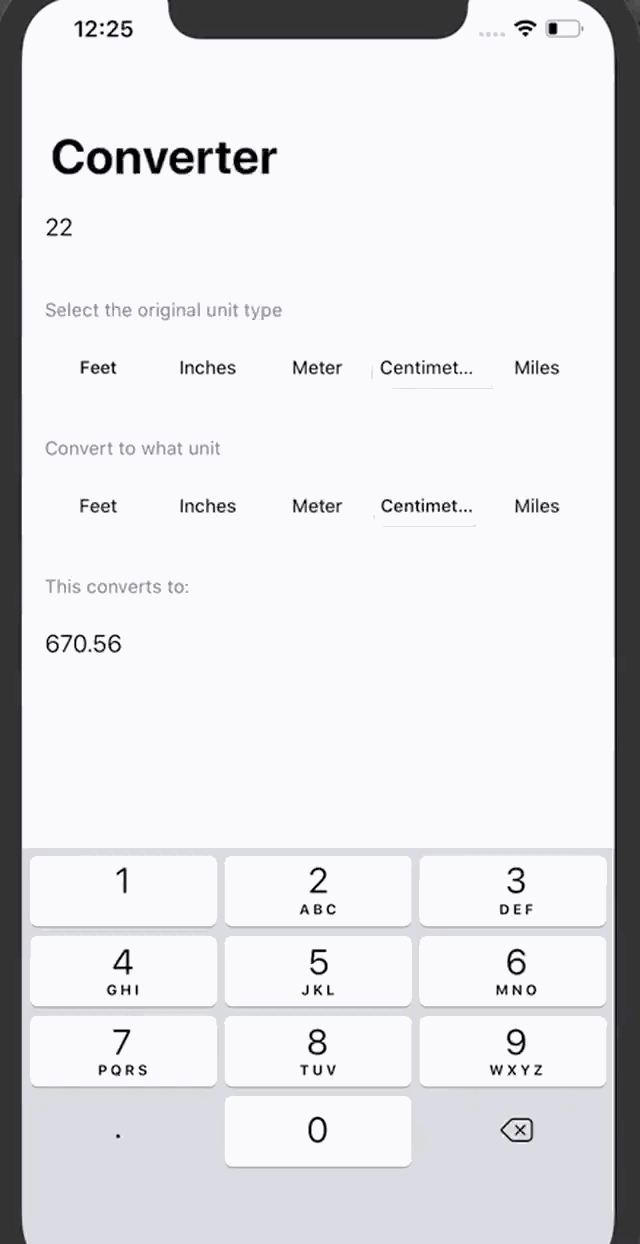
I built a converter application that uses the Measurement class in Swift to convert what the user gives into another length unit. So if they put in 500 inches and want to know the amount of meters, the application will give them that information. The first step in that process is to take what they input, the original amount, and convert it to a the Measurement class, using the type they’ve selected. I created a switch statement to get that done.
var measurement : Measurement<UnitLength>{
let original = Double(amount) ?? 0
switch units[originalUnit] {
case "Feet":
return Measurement(value: original, unit: UnitLength.feet)
case "Inches":
return Measurement(value: original, unit: UnitLength.inches)
case "Miles":
return Measurement(value: original, unit: UnitLength.miles)
case "Meter":
return Measurement(value: original, unit: UnitLength.meters)
default:
return Measurement(value: original, unit: UnitLength.centimeters)
}
}
The next step is to take that Measurement class and run the convert function on it, to the type the user selects. If they want inches turning into Centimeters, then that’s what this next bit of code will do.
var convertedUnitValue: Measurement<UnitLength> {
switch units[selectedUnit] {
case "Feet":
return measurement.converted(to: UnitLength.feet)
case "Inches":
return measurement.converted(to: UnitLength.inches)
case "Miles":
return measurement.converted(to: UnitLength.miles)
case "Meter":
return measurement.converted(to: UnitLength.meters)
default:
return measurement.converted(to: UnitLength.centimeters)
}
}
Both of these code snippets are computed properties and for that reason they need to be of type Measurement<UnitLength>. It took me a bit to figure that part out, as this wasn’t a tutorial but our first pass at an app on our own. This is because they’re actually being assigned that type from the Switch statement. At first, I thought they might be doubles but they’re not returning the .value, but the full measurement. They also do not use the -> return syntax for type, because it’s assigning to that variable, not really returning it.